getimagesize
GET physical information about image files.
This function does not require the
GD librarie.
This function returns, directly, the size of any supported given image file and return the dimensions along with the file type and a height/width text string to be used inside a normal HTML IMG tag and the correspondent HTTP content type.
Additionally can also return some more information in $imageinfo parameter passed as reference.
This function expects $filename to be a valid image file.
If a non-image file is supplied, it may be incorrectly detected as an image and the function will return successfully, but the array may contain nonsensical values.
You should not use this function to check that a given file is a valid image; there are other functions to do this.
Some image files like JPC and JP2 are capable of having components with different bit depths.
In this case, the value for bits is the highest bit depth encountered.
Also, JP2 files may contain multiple JPEG 2000 codestreams.
In this case, this function returns the values for the first codestream it encounters in the root of the file.
The information about icons are retrieved from the icon with the highest bitrate.
In the GIF images with one or more frames, where each frame may only occupy part of the image; the size reported by this function is the overal size, that is readed by the logical screen descriptor.
$filename can reference a local file or, (configuration permitting), a remote file using one of the supported stream wrappers:
$imageinfo is the optional parameter that allows to extract some extended information from the image file.
There are other functions which display such information in more detail, if available, in JPEG files.
<?php
array|false getimagesize(string $filename, array &$image_info = null)
where,
$filename = The file to retrieve information about
&$image_info = The extended information from the image file
( PASSED as reference )
?>
$filename
The path to the image file.
STREAM WRAPPERS |
MEANING |
file:// |
LOCAL File System |
http:// |
URL HTTP |
ftp:// |
URL FTP |
php:// |
I/O streams |
zlib:// |
Data Compression stream |
data:// |
DATA (RFC-2397) |
glob:// |
Find Filenames |
phar:// |
PHP Archive |
ssh2:// |
Secure Shell 2 |
rar:// |
RAR |
ogg:// |
Audio |
expect:// |
Interaction of stream processes |
ed48 |
&$image_info
The metadata elements, if they exist in the tested image file.
EXERCISE
<?php
$arr_img01 = [ 'E 01 01.gif',
'E 01 02.jpg',
'E 01 03.png',
'E 01 06.bmp',
'E 01 17.ico',
'E 01 18.webp' ];
foreach($arr_img01 as $img01)
{
$get_info01 = getimagesize('img/' . $img01);
echo $img01;
$wd = $get_info01['0'];
echo '<br><br><img src="' . 'img/' .
$img01 . '" alt="' . $img01 .
'" width="' . $wd . '"%" title="' . $img01 . '"><br>';
echo '<pre>';
var_dump($get_info01);
echo '</pre><br><br><br>';
}
?>
RESULT E 01 01.gif
array(7) {
[0]=>
int(400)
[1]=>
int(265)
[2]=>
int(1)
[3]=>
string(24) "width="400" height="265""
["bits"]=>
int(8)
["channels"]=>
int(3)
["mime"]=>
string(9) "image/gif"
}
E 01 02.jpg
array(7) {
[0]=>
int(400)
[1]=>
int(265)
[2]=>
int(2)
[3]=>
string(24) "width="400" height="265""
["bits"]=>
int(8)
["channels"]=>
int(3)
["mime"]=>
string(10) "image/jpeg"
}
E 01 03.png
array(6) {
[0]=>
int(400)
[1]=>
int(265)
[2]=>
int(3)
[3]=>
string(24) "width="400" height="265""
["bits"]=>
int(8)
["mime"]=>
string(9) "image/png"
}
E 01 06.bmp
array(6) {
[0]=>
int(400)
[1]=>
int(265)
[2]=>
int(6)
[3]=>
string(24) "width="400" height="265""
["bits"]=>
int(24)
["mime"]=>
string(9) "image/bmp"
}
E 01 17.ico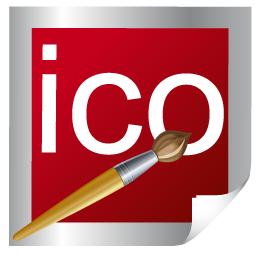
array(6) {
[0]=>
int(16)
[1]=>
int(16)
[2]=>
int(17)
[3]=>
string(22) "width="16" height="16""
["bits"]=>
int(32)
["mime"]=>
string(24) "image/vnd.microsoft.icon"
}
E 01 18.webp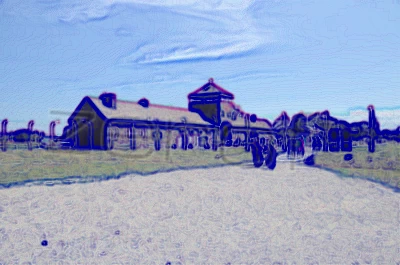
array(6) {
[0]=>
int(400)
[1]=>
int(265)
[2]=>
int(18)
[3]=>
string(24) "width="400" height="265""
["bits"]=>
int(8)
["mime"]=>
string(10) "image/webp"
}
EXERCISE
<?php
$img02 = 'E 01.jpg';
$get_info02 = getimagesize('img/' . $img02);
echo $img02;
$wd = $get_info02['0'];
echo '<br><br><img src="' . 'img/' .
$img02 . '" alt="' . $img02 .
'" width="300" title="' . $img02 . '"><br>';
echo '<pre>';
var_dump($get_info02);
echo '</pre><br>';
?>
RESULT E 01.jpg
array(7) {
[0]=>
int(4032)
[1]=>
int(3024)
[2]=>
int(2)
[3]=>
string(26) "width="4032" height="3024""
["bits"]=>
int(8)
["channels"]=>
int(3)
["mime"]=>
string(10) "image/jpeg"
}